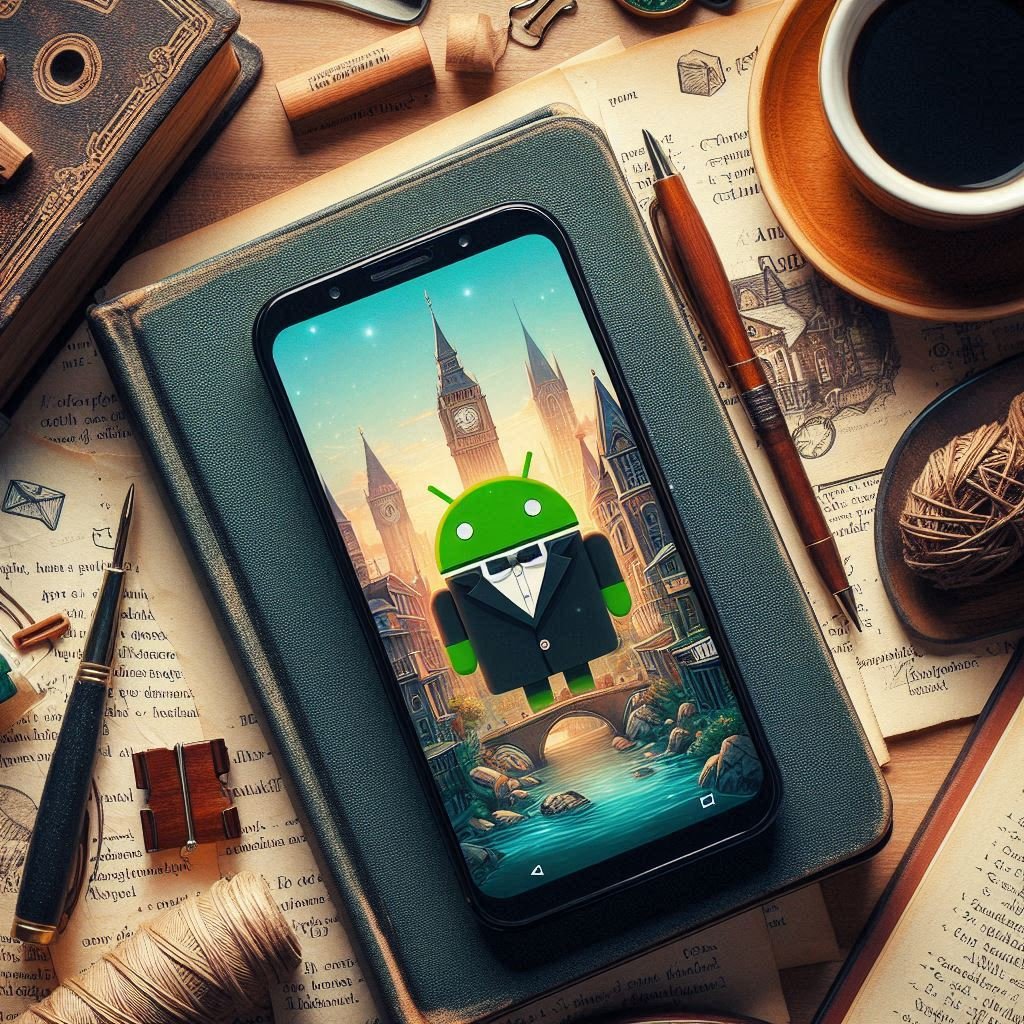
Understanding TextView in Android App Development with Java
In Android app development, displaying text on the user interface is one of the most fundamental aspects of creating engaging, informative apps. TextView
is the widget in Android that serves this purpose, enabling developers to display, format, and style text within the app interface. This article explores the TextView
widget, its properties, and practical usage in Android app development with Java.
1. What is TextView?
A TextView
in Android is a user interface element that allows developers to display static or dynamic text to users. It is an extremely versatile widget, commonly used for displaying labels, instructions, messages, or any other textual content within an app. It can display a single line of text or multiple lines, and its appearance can be customized extensively.
2. Adding TextView to an XML Layout
To use a TextView
, you need to add it to your XML layout file. Here’s a simple example of how to add a TextView
:
<TextView
android:id="@+id/textViewExample"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello, World!"
android:textSize="18sp"
android:textColor="#000000" />
In this code snippet:
android:id
assigns an ID to theTextView
for referencing it in Java code.android:layout_width
andandroid:layout_height
specify the dimensions, often set towrap_content
to fit the text.android:text
sets the displayed text.android:textSize
sets the text size.android:textColor
defines the color of the text.
3. Working with TextView in Java
After defining a TextView
in XML, you can control its behavior and modify it in your Java code. To reference and modify TextView
, follow these steps:
- Initialize the TextView: Find it by ID in the
Activity
class. - Set Text: Use
setText()
to update its content dynamically.
Here’s an example in Java:
import android.os.Bundle;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Initialize TextView by finding it by ID
TextView textViewExample = findViewById(R.id.textViewExample);
// Set new text programmatically
textViewExample.setText("Welcome to Android Development with Java!");
}
}
4. Key Properties of TextView
The TextView
widget comes with a variety of properties that allow customization:
- Text Size: Set with
android:textSize
in XML orsetTextSize()
in Java. - Text Color: Use
android:textColor
in XML orsetTextColor()
in Java. - Font Style: Control font style with
android:textStyle
for options like bold or italic. - Text Alignment: Center or align text with
android:gravity
orandroid:textAlignment
. - Max Lines and Ellipsis: Control the number of lines and handle overflow text with
android:maxLines
andandroid:ellipsize
.
Example:
<TextView
android:id="@+id/textViewStyled"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Styled TextView"
android:textSize="20sp"
android:textColor="#FF5722"
android:textStyle="bold"
android:gravity="center"
android:ellipsize="end"
android:maxLines="1"/>
5. Using Spans for Text Styling
Android supports the use of spans to apply different styles to portions of text within a TextView
. With spans, you can make parts of the text bold, italicized, or even clickable.
Example with SpannableString
:
import android.graphics.Color;
import android.os.Bundle;
import android.text.Spannable;
import android.text.SpannableString;
import android.text.style.ForegroundColorSpan;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
TextView textView = findViewById(R.id.textViewExample);
SpannableString spannable = new SpannableString("Welcome to Android Development!");
// Apply color span to a portion of text
spannable.setSpan(new ForegroundColorSpan(Color.RED), 11, 18, Spannable.SPAN_EXCLUSIVE_EXCLUSIVE);
textView.setText(spannable);
}
}
This example changes the color of the text “Android” to red while leaving the rest unchanged.
6. Clickable Text in TextView
If you want to make part of the text in a TextView
clickable, use Spannable
to apply a ClickableSpan
. This is especially useful for creating links within text.
Example:
import android.os.Bundle;
import android.text.SpannableString;
import android.text.Spanned;
import android.text.method.LinkMovementMethod;
import android.text.style.ClickableSpan;
import android.view.View;
import android.widget.TextView;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
TextView textView = findViewById(R.id.textViewExample);
SpannableString spannable = new SpannableString("Click here to learn more about Android development!");
ClickableSpan clickableSpan = new ClickableSpan() {
@Override
public void onClick(View widget) {
Toast.makeText(MainActivity.this, "Clicked!", Toast.LENGTH_SHORT).show();
}
};
spannable.setSpan(clickableSpan, 6, 10, Spanned.SPAN_EXCLUSIVE_EXCLUSIVE);
textView.setText(spannable);
textView.setMovementMethod(LinkMovementMethod.getInstance());
}
}
In this code, “here” is clickable, and clicking it displays a toast message.
7. Using HTML in TextView
To display HTML content in a TextView
, Android offers the Html.fromHtml()
method. This is useful for rendering HTML content in applications such as blogs or news feeds.
Example:
import android.os.Bundle;
import android.text.Html;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
TextView textView = findViewById(R.id.textViewExample);
String html = "<h2>Android Development</h2><p>This is a TextView with HTML content.</p>";
textView.setText(Html.fromHtml(html));
}
}
This code displays HTML-formatted text in a TextView
.
8. Advanced Styling with Custom Fonts
To further personalize a TextView
, you can use custom fonts. Here’s a quick overview:
- Place the font file in
res/font
(e.g.,res/font/myfont.ttf
). - Reference it in XML or set it programmatically.
Example:
<TextView
android:id="@+id/textViewCustomFont"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:fontFamily="@font/myfont"
android:text="Custom Font TextView" />
9. TextView Best Practices
- Use Spans for Inline Styling: Apply styles like bold, color, or underline to parts of text with spans for better control and readability.
- Limit Lines for Labels: Use
android:maxLines
andandroid:ellipsize
to handle overflow in labels and messages. - Optimize for Performance: Reuse
TextView
widgets, especially in lists and grids, to reduce memory consumption.
Conclusion
TextView
is one of the most essential widgets in Android development, allowing developers to display and style text content effectively. By mastering its properties, customization options, and capabilities for spans, HTML, and custom fonts, you can create dynamic, user-friendly text displays in your Android apps.