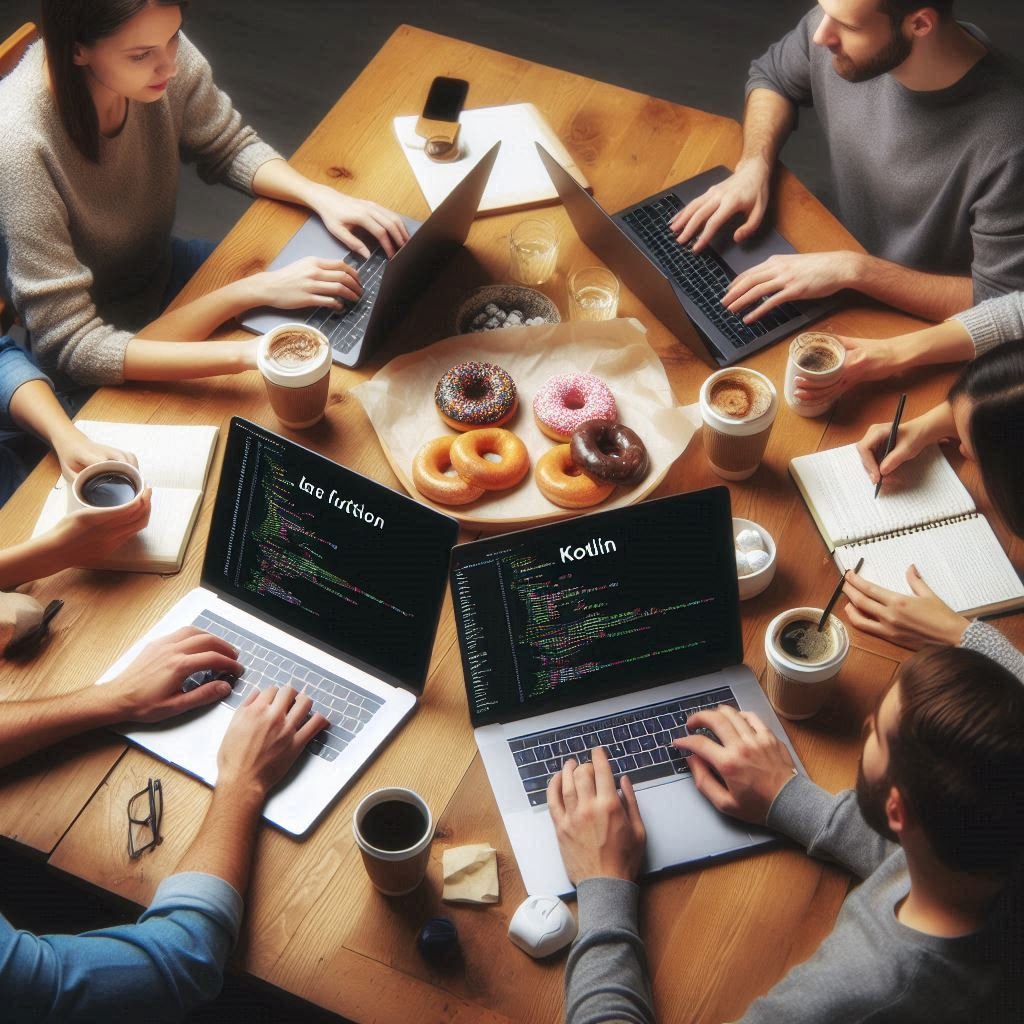
Functions in Kotlin play a central role in organizing code and executing logic in a structured way. This guide covers everything you need to know about functions in Kotlin, from basic syntax to advanced usage.
Course Outline
- Introduction to Kotlin Functions
- What are functions?
- The purpose and benefits of using functions
- Basic Function Syntax
- Declaring functions
- Function parameters and arguments
- Returning values
- Function Types
- Top-level functions
- Member functions
- Local functions
- Extension functions
- Named and Default Arguments
- Using named arguments
- Defining and using default parameter values
- Single-Expression Functions
- Simplifying functions with single-expression syntax
- Higher-Order Functions and Lambdas
- Introduction to higher-order functions
- Using lambda expressions
- Inline Functions
- Understanding and using inline functions for performance
- Recursion in Kotlin
- Recursive functions
- Tail recursion optimization
- Examples and Exercises
1. Introduction to Kotlin Functions
In Kotlin, a function is a reusable block of code designed to perform a particular task. Functions allow you to break your program into smaller, manageable parts and promote code reusability, making it easier to maintain and understand.
2. Basic Function Syntax
A function in Kotlin is defined using the fun
keyword, followed by a name, parameters (optional), and a return type (optional).
fun functionName(parameter1: Type, parameter2: Type): ReturnType {
// Function body
return result
}
Example of a Simple Function
fun greet(name: String): String {
return "Hello, $name!"
}
println(greet("Alice")) // Output: Hello, Alice!
In this example:
greet
is the function name.name
is a parameter of typeString
.String
is the return type of the function.
Functions without Parameters
fun sayHello() {
println("Hello!")
}
sayHello() // Output: Hello!
Functions without Return Values
Functions that don’t return a value have a return type of Unit
, which can be omitted.
fun printMessage(message: String): Unit {
println(message)
}
3. Function Types
Kotlin supports various types of functions:
- Top-level functions: Defined outside of any class.
- Member functions: Defined within a class or object.
- Local functions: Defined inside another function.
- Extension functions: Allow you to extend the functionality of existing classes.
Example of a Top-Level Function
fun topFunction() {
println("This is a top-level function.")
}
Example of a Member Function
class Person(val name: String) {
fun introduce() {
println("Hi, I'm $name.")
}
}
Example of a Local Function
fun outerFunction() {
fun innerFunction() {
println("This is a local function.")
}
innerFunction()
}
Example of an Extension Function
fun String.addExclamation(): String {
return this + "!"
}
println("Hello".addExclamation()) // Output: Hello!
4. Named and Default Arguments
Kotlin functions support named arguments, allowing you to specify which argument goes to which parameter, and default arguments, which provide a fallback value if no argument is supplied.
Using Named Arguments
fun introduce(name: String, age: Int) {
println("My name is $name and I am $age years old.")
}
introduce(age = 30, name = "Alice")
Using Default Arguments
fun greet(name: String = "Guest") {
println("Hello, $name!")
}
greet() // Output: Hello, Guest!
greet("Alice") // Output: Hello, Alice!
5. Single-Expression Functions
If a function consists of a single expression, you can simplify it with single-expression syntax by using =
instead of curly braces {}
.
fun square(x: Int): Int = x * x
6. Higher-Order Functions and Lambdas
Kotlin supports higher-order functions, which are functions that take other functions as parameters or return them. This feature enables Kotlin to support functional programming constructs.
Example of a Higher-Order Function
fun calculate(x: Int, y: Int, operation: (Int, Int) -> Int): Int {
return operation(x, y)
}
val result = calculate(3, 4, { a, b -> a + b })
println(result) // Output: 7
Using Lambda Expressions
Lambda expressions are anonymous functions that can be used as function literals.
val multiply = { a: Int, b: Int -> a * b }
println(multiply(3, 4)) // Output: 12
7. Inline Functions
Inline functions can improve performance by avoiding function call overhead in higher-order functions. To make a function inline, use the inline
keyword.
inline fun performOperation(x: Int, y: Int, operation: (Int, Int) -> Int): Int {
return operation(x, y)
}
8. Recursion in Kotlin
Recursion is when a function calls itself to solve a smaller instance of the same problem.
Example of a Recursive Function
fun factorial(n: Int): Int {
return if (n == 1) 1 else n * factorial(n - 1)
}
println(factorial(5)) // Output: 120
Tail-Recursive Functions
Kotlin supports tail recursion, which optimizes recursive functions. Use the tailrec
modifier to indicate that a function is tail-recursive.
tailrec fun tailFactorial(n: Int, result: Int = 1): Int {
return if (n == 1) result else tailFactorial(n - 1, result * n)
}
9. Examples and Exercises
Example 1: A Function to Calculate the Average of Two Numbers
fun average(x: Double, y: Double): Double {
return (x + y) / 2
}
println(average(4.0, 6.0)) // Output: 5.0
Exercise 2: Create a Function to Check if a Number is Even
fun isEven(number: Int): Boolean {
return number % 2 == 0
}
println(isEven(4)) // Output: true
println(isEven(5)) // Output: false
Exercise 3: Higher-Order Function for Applying a Discount
Create a higher-order function that applies a discount to a given price.
fun applyDiscount(price: Double, discount: (Double) -> Double): Double {
return discount(price)
}
val discountedPrice = applyDiscount(100.0) { it * 0.9 }
println(discountedPrice) // Output: 90.0
Conclusion
Kotlin’s function features offer a rich and flexible way to structure and optimize your code. Whether you are working with basic functions, higher-order functions, or recursion, mastering Kotlin functions allows you to write cleaner, more efficient, and more modular code. By practicing the exercises provided, you’ll develop a deeper understanding of how to utilize Kotlin functions effectively in various scenarios.
With this course, you now have a solid foundation for working with functions in Kotlin. Practice regularly to become proficient in creating, using, and optimizing functions in your Kotlin projects.