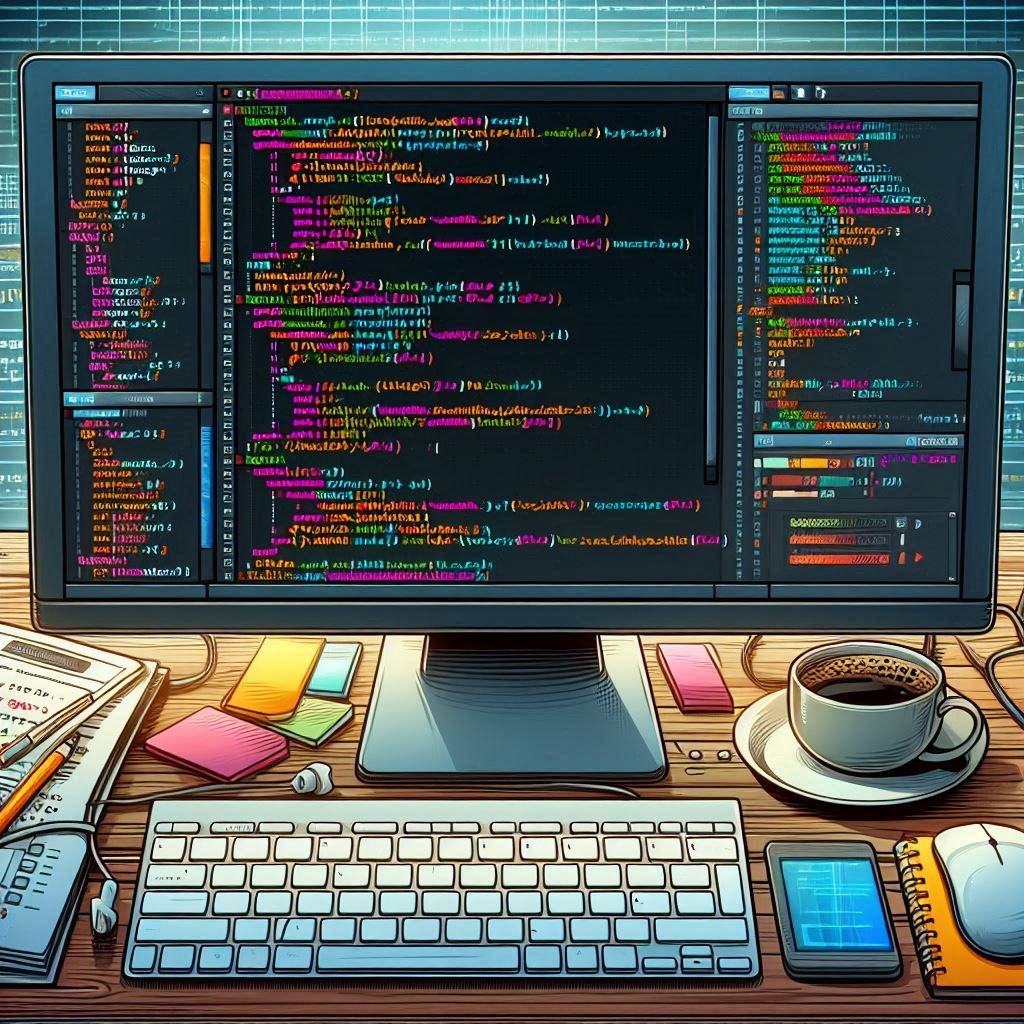
Java Strings: A Complete Guide
In Java, Strings are one of the most commonly used objects, and they represent sequences of characters. Java Strings are widely used for storing and manipulating textual data, such as names, messages, and other forms of content. Understanding how to work with strings is essential for Java developers.
This article will provide a comprehensive guide to Java Strings, including their creation, common operations, and best practices. It will also include exercises to help you practice your knowledge of working with Strings in Java.
What is a String in Java?
In Java, a String is an object that represents a sequence of characters. Strings are immutable in Java, meaning once created, their values cannot be changed. If you modify a String, a new String object is created.
String Class
Java provides the String
class in the java.lang
package to work with string data. Since String
is a class, it has various methods that you can use to perform operations on string objects.
Creating Strings in Java
Strings in Java can be created in two ways:
- String Literal You can create a string using double quotes
"
.String str = "Hello, Java!";
- Using the
new
Keyword You can also create a string using thenew
keyword.String str = new String("Hello, Java!");
In both cases, the string content is the same, but using the new
keyword explicitly creates a new object in memory.
String Operations in Java
Java provides a wide variety of methods for manipulating strings. Some of the most commonly used operations are described below.
1. String Length
The length()
method returns the number of characters in the string.
String str = "Hello, Java!";
int length = str.length(); // Returns 13
System.out.println("Length: " + length);
2. Concatenation
You can combine two or more strings using the +
operator or the concat()
method.
String str1 = "Hello, ";
String str2 = "Java!";
String result = str1 + str2; // Using + operator
// OR
result = str1.concat(str2); // Using concat() method
System.out.println(result); // Prints: Hello, Java!
3. Substring
The substring()
method returns a part of the string, starting from a specified index to the end, or from a start index to an end index.
String str = "Hello, Java!";
String substr = str.substring(7); // From index 7 to the end
System.out.println(substr); // Prints: Java!
substr = str.substring(0, 5); // From index 0 to index 5 (excluding 5)
System.out.println(substr); // Prints: Hello
4. String Comparison
The equals()
method checks if two strings are equal, and the compareTo()
method compares two strings lexicographically.
String str1 = "Hello";
String str2 = "hello";
String str3 = "Hello";
boolean result = str1.equals(str2); // Compares strings for equality (case-sensitive)
System.out.println("Are str1 and str2 equal? " + result); // Prints: false
result = str1.equals(str3); // Compares strings for equality
System.out.println("Are str1 and str3 equal? " + result); // Prints: true
int comparison = str1.compareTo(str2); // Compares lexicographically
System.out.println("Comparison result: " + comparison); // Prints a negative number since 'H' < 'h'
5. Converting to Uppercase and Lowercase
The toUpperCase()
and toLowerCase()
methods are used to convert the string to uppercase and lowercase respectively.
String str = "Hello, Java!";
String upper = str.toUpperCase();
String lower = str.toLowerCase();
System.out.println(upper); // Prints: HELLO, JAVA!
System.out.println(lower); // Prints: hello, java!
6. Trimming Whitespaces
The trim()
method removes any leading or trailing whitespace from the string.
String str = " Hello, Java! ";
String trimmed = str.trim();
System.out.println(trimmed); // Prints: Hello, Java!
7. Checking String Containment
The contains()
method checks if a particular sequence of characters exists in the string.
String str = "Hello, Java!";
boolean result = str.contains("Java");
System.out.println(result); // Prints: true
Commonly Used String Methods in Java
Method | Description |
---|---|
length() | Returns the length of the string. |
equals() | Compares two strings for equality. |
compareTo() | Compares two strings lexicographically. |
toLowerCase() | Converts the string to lowercase. |
toUpperCase() | Converts the string to uppercase. |
substring() | Extracts a part of the string. |
trim() | Removes leading and trailing whitespaces. |
contains() | Checks if the string contains a sequence of characters. |
indexOf() | Returns the index of the first occurrence of a character. |
charAt() | Returns the character at a specific index. |
replace() | Replaces a specified character or substring with another. |
Exercises on Java Strings
Exercise 1: String Concatenation
Write a program that takes two strings as input and concatenates them using both the +
operator and the concat()
method. Print both results.
Exercise 2: Substring Extraction
Create a program that extracts the first three characters of a string. Test this by using different strings and printing the result.
Exercise 3: String Comparison
Write a program that compares two strings input by the user. Print whether the strings are equal or not using both the equals()
and compareTo()
methods.
Exercise 4: Case Conversion
Write a program that converts a given string into uppercase and lowercase. Display both results.
Exercise 5: String Containment
Write a program that checks if a given string contains a particular word (e.g., “Java”) and prints whether it is found or not.
Exercise 6: Trimming and Length
Write a program that takes a string input with extra spaces at the beginning and end. Remove the spaces and print the length of the trimmed string.
Conclusion
Strings are one of the most powerful and frequently used data types in Java. The String
class offers a wide range of methods to perform various operations on string data, such as concatenation, comparison, and manipulation. Understanding how to efficiently use strings is crucial for Java developers.
By completing the exercises, you will gain hands-on experience with string operations in Java and develop a deeper understanding of how to handle and manipulate string data effectively in your applications.