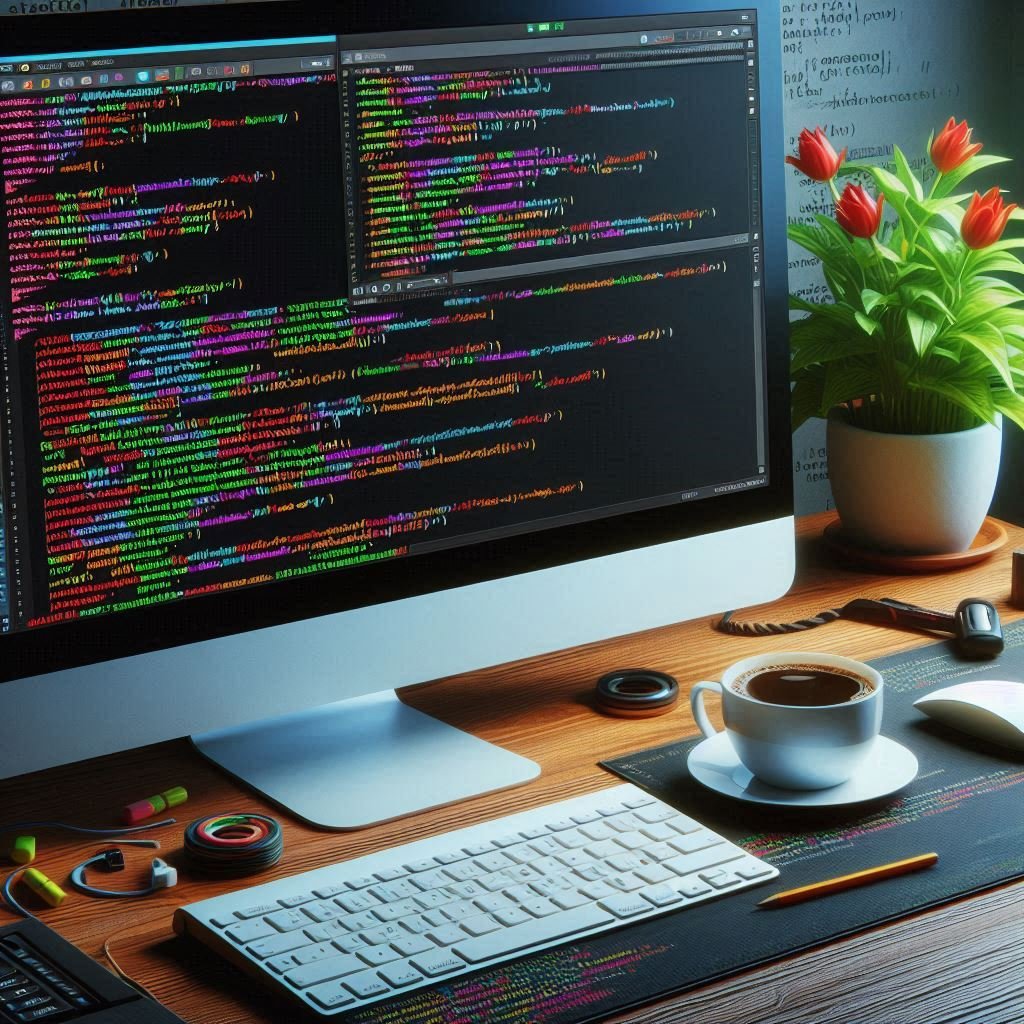
Java Regular Expressions: A Complete Guide with Examples and Exercises
A regular expression (often abbreviated as regex) is a sequence of characters that defines a search pattern. Regular expressions are widely used for searching, editing, and manipulating text, and they are an essential tool for handling string-related tasks in Java.
In this article, we will explore how Java handles regular expressions, provide a detailed explanation of regex syntax, discuss common use cases, and present exercises to help you practice your skills in using Java regular expressions.
Table of Contents
- Introduction to Regular Expressions
- Java Regex Syntax
- Using Regular Expressions in Java
- Common Regex Use Cases
- Regex Flags
- Matching, Replacing, and Splitting with Regex
- Exercise
- Conclusion
1. Introduction to Regular Expressions
A regular expression is a pattern used to match character combinations in strings. In Java, regular expressions are supported via the java.util.regex
package, which contains the Pattern
and Matcher
classes that enable working with regex.
Regular expressions allow you to perform complex searches and manipulations on strings. They are commonly used for tasks such as:
- Validating input (e.g., email addresses, phone numbers)
- Searching and replacing text
- Splitting strings based on patterns
For example, a regex pattern \d{3}-\d{2}-\d{4}
can be used to match a Social Security number in the format 123-45-6789
.
2. Java Regex Syntax
Java regular expressions follow a specific syntax that includes literal characters and metacharacters. Below is an overview of the basic components of Java regular expressions:
1. Literal Characters
Literal characters match themselves. For example, the regex hello
matches the string “hello”.
2. Metacharacters
Metacharacters are symbols that control how the regex is matched:
.
(dot): Matches any single character except newline.^
: Matches the beginning of a string.$
: Matches the end of a string.[]
: A character class, matches any single character within the brackets.|
: Acts as a logical OR between two patterns.()
: Groups patterns together.*
: Matches 0 or more occurrences of the preceding element.+
: Matches 1 or more occurrences of the preceding element.?
: Matches 0 or 1 occurrence of the preceding element.\d
: Matches any digit (equivalent to[0-9]
).\w
: Matches any word character (alphanumeric or underscore).\s
: Matches any whitespace character (space, tab, newline).{n,m}
: Matches betweenn
andm
occurrences of the preceding element.
Examples:
\d{3}
: Matches exactly 3 digits (e.g., “123”).\w+
: Matches one or more word characters (e.g., “Hello123”).\s*
: Matches zero or more whitespace characters.
3. Using Regular Expressions in Java
In Java, you work with regular expressions using the Pattern
and Matcher
classes.
1. Pattern Class
The Pattern
class represents a compiled regular expression. You can create a Pattern
object using the Pattern.compile()
method.
import java.util.regex.*;
public class RegexExample {
public static void main(String[] args) {
// Compile a regular expression
Pattern pattern = Pattern.compile("a*b");
// Create a matcher for the pattern
Matcher matcher = pattern.matcher("aaab");
// Check if the pattern matches
if (matcher.matches()) {
System.out.println("Pattern matches!");
} else {
System.out.println("Pattern does not match.");
}
}
}
2. Matcher Class
The Matcher
class is used to perform matching operations on a given input string. Common methods include:
matches()
: Attempts to match the entire string against the regex pattern.find()
: Searches for the next occurrence of the pattern in the string.replaceAll()
: Replaces all occurrences of the pattern with a given replacement.split()
: Splits the string into an array of substrings based on the regex.
Example of using find()
:
Pattern pattern = Pattern.compile("\\d+");
Matcher matcher = pattern.matcher("There are 123 apples and 45 oranges.");
while (matcher.find()) {
System.out.println("Found number: " + matcher.group());
}
Output:
Found number: 123
Found number: 45
4. Common Regex Use Cases
1. Validating Email Address
A regex pattern can be used to validate email addresses, ensuring that the format is correct.
Example pattern:^[a-zA-Z0-9_+&*-]+(?:\\.[a-zA-Z0-9_+&*-]+)*@(?:[a-zA-Z0-9-]+\\.)+[a-zA-Z]{2,7}$
Pattern emailPattern = Pattern.compile("^[a-zA-Z0-9_+&*-]+(?:\\.[a-zA-Z0-9_+&*-]+)*@(?:[a-zA-Z0-9-]+\\.)+[a-zA-Z]{2,7}$");
Matcher matcher = emailPattern.matcher("test@example.com");
if (matcher.matches()) {
System.out.println("Valid email.");
} else {
System.out.println("Invalid email.");
}
2. Phone Number Validation
A regex pattern can validate phone numbers in different formats.
Example pattern for a US phone number:^(\\+1)?\\(?\\d{3}\\)?[-.\\s]?\\d{3}[-.\\s]?\\d{4}$
Pattern phonePattern = Pattern.compile("^(\\+1)?\\(?\\d{3}\\)?[-.\\s]?\\d{3}[-.\\s]?\\d{4}$");
Matcher matcher = phonePattern.matcher("(123) 456-7890");
if (matcher.matches()) {
System.out.println("Valid phone number.");
} else {
System.out.println("Invalid phone number.");
}
5. Regex Flags
Regex flags allow you to modify the behavior of the pattern matching process. Common flags include:
Pattern.CASE_INSENSITIVE
: Makes the pattern matching case-insensitive.Pattern.DOTALL
: Allows the dot (.
) to match newline characters.Pattern.MULTILINE
: Allows^
and$
to match at the start and end of each line, not just the entire string.
Example of using a flag:
Pattern pattern = Pattern.compile("hello", Pattern.CASE_INSENSITIVE);
Matcher matcher = pattern.matcher("HELLO");
if (matcher.matches()) {
System.out.println("Pattern matches.");
} else {
System.out.println("Pattern does not match.");
}
6. Matching, Replacing, and Splitting with Regex
1. Matching
Use the matches()
method to check if the entire string matches the regular expression.
Pattern pattern = Pattern.compile("\\d{4}");
Matcher matcher = pattern.matcher("2024");
if (matcher.matches()) {
System.out.println("Valid year.");
}
2. Replacing
You can replace all occurrences of the pattern with a replacement string using replaceAll()
.
String input = "abc 123 def 456";
String result = input.replaceAll("\\d+", "number");
System.out.println(result); // Output: abc number def number
3. Splitting
The split()
method splits the input string into an array of substrings based on the regex pattern.
String input = "apple,banana,grape";
String[] fruits = input.split(",");
for (String fruit : fruits) {
System.out.println(fruit);
}
7. Exercise
Exercise 1: Email Validation
Write a program that accepts a user’s email address and checks whether it is valid using a regex pattern.
Exercise 2: Phone Number Formatting
Write a program that takes a phone number input and ensures it is in the format (XXX) XXX-XXXX
. If the input does not match, prompt the user to enter a valid phone number.
Solution for Exercise 1:
import java.util.regex.*;
public class EmailValidation {
public static void main(String[] args) {
String email = "user@example.com";
Pattern pattern = Pattern.compile("^[a-zA-Z0-9_+&*-]+(?:\\.[a-zA-Z0-9_+&*-]+)*@(?:[a-zA-Z0-9-]+\\.)+[a-zA-Z]{2,7}$");
Matcher matcher = pattern.matcher(email);
if (matcher.matches()) {
System.out.println("Valid email.");
} else {
System.out.println("Invalid email.");
}
}
}
Solution for Exercise 2:
import java.util.regex.*;
public class PhoneNumberValidation {
public static void main(String[] args) {
String phoneNumber = "(123) 456-7890";
Pattern pattern = Pattern.compile("^(\\+1
)?\\(?\\d{3}\\)?[-.\\s]?\\d{3}[-.\\s]?\\d{4}$");
Matcher matcher = pattern.matcher(phoneNumber);
if (matcher.matches()) {
System.out.println("Valid phone number.");
} else {
System.out.println("Invalid phone number. Please enter in the format (XXX) XXX-XXXX.");
}
}
}
8. Conclusion
Regular expressions are a powerful tool in Java for pattern matching, searching, and manipulating strings. Mastering regular expressions is essential for tasks like input validation, data parsing, and text processing. By understanding the syntax and common use cases, and practicing with the exercises, you will be able to efficiently use regular expressions in your Java programs.