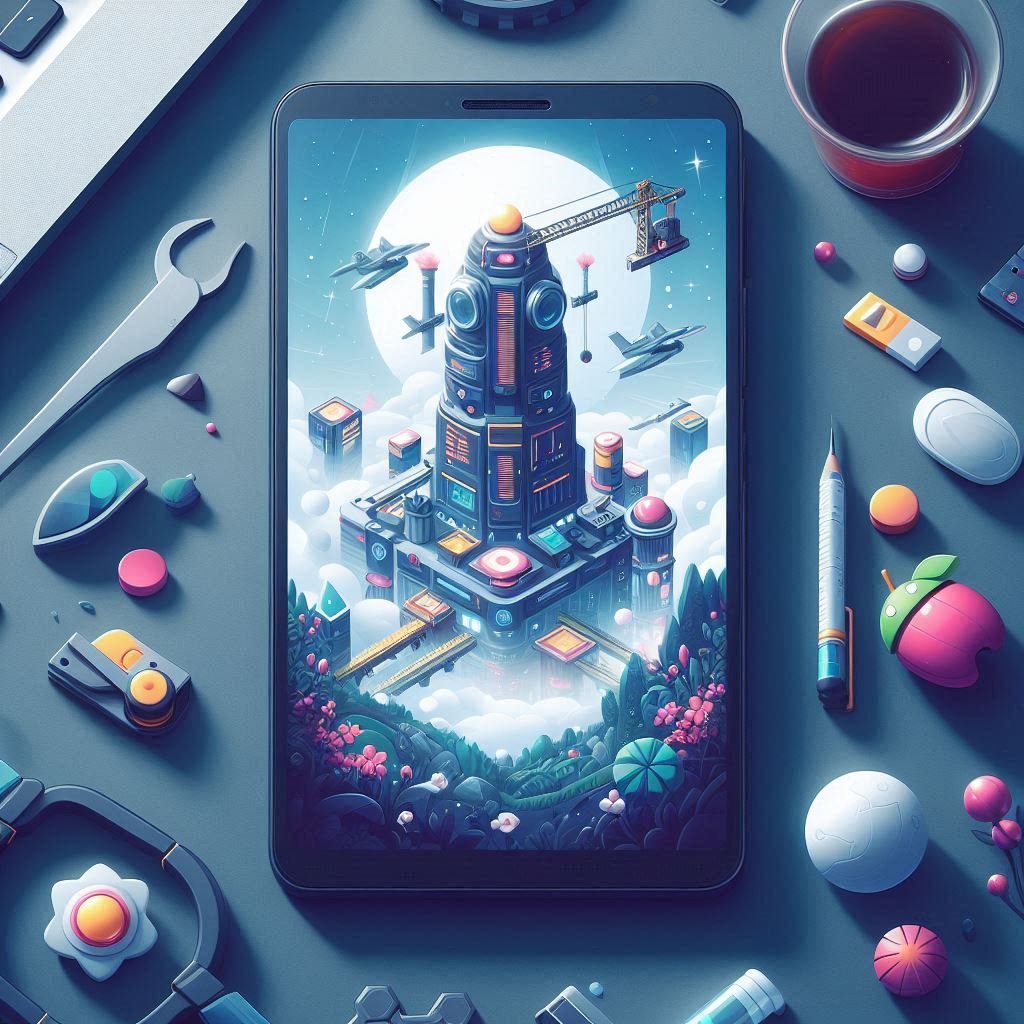
Gradle Build in Android App Development: A Complete Guide
In Android app development, Gradle is an essential tool for automating the build process. It is a powerful build automation system that is widely used in Android projects to manage dependencies, build configurations, and packaging. Gradle works alongside Android Studio to help developers build, test, and deploy their applications efficiently.
This article explores Gradle builds in Android app development, explaining how Gradle is used in Android projects, how to configure it, and how it simplifies the development process.
What is Gradle?
Gradle is an open-source build automation system that supports multi-language development, but it is most widely known for its use in Android development. Gradle allows developers to define a custom build process and automate repetitive tasks, such as:
- Compiling code
- Managing dependencies
- Packaging apps
- Running tests
- Generating documentation
In Android, Gradle is used to manage the entire app lifecycle, from compiling the source code to generating the APK or AAB (Android App Bundle) for distribution. Gradle also makes it easy to configure different build variants (e.g., debug and release versions) and manage dependencies from external libraries.
How Gradle Works in Android App Development
In Android app development, Gradle is integrated into Android Studio and acts as the backbone for the build system. Android Studio uses Gradle to compile the app, resolve dependencies, package resources, and create APKs or AABs. Gradle uses configuration files, such as build.gradle
, to define the build process.
Gradle Files in Android
There are two main build.gradle
files in an Android project:
- Project-level
build.gradle
: This file defines configuration options that apply to the entire project, such as the Gradle plugin version, repositories, and global dependencies. - App-level
build.gradle
: This file defines the configuration specific to a single module (e.g., the app module), such as dependencies, build types, product flavors, and other settings.
Each module in an Android project can have its own build.gradle
file, especially if you’re working with multiple modules in a larger project.
Example of Project-Level build.gradle
// Top-level build.gradle file, in the root directory of the project
buildscript {
repositories {
google() // Google's repository
mavenCentral() // Maven Central repository for dependencies
}
dependencies {
classpath 'com.android.tools.build:gradle:7.3.0' // Android Gradle Plugin
classpath 'org.jetbrains.kotlin:kotlin-gradle-plugin:1.7.0' // Kotlin plugin (if using Kotlin)
}
}
allprojects {
repositories {
google()
mavenCentral()
}
}
Example of App-Level build.gradle
// app-level build.gradle file (usually located in the "app" directory)
plugins {
id 'com.android.application' // Android application plugin
id 'org.jetbrains.kotlin.android' // Kotlin plugin (if using Kotlin)
}
android {
compileSdkVersion 33 // Specifies the version of the SDK to compile against
defaultConfig {
applicationId "com.example.myapp"
minSdkVersion 21 // Minimum supported SDK version
targetSdkVersion 33 // Target SDK version
versionCode 1 // Version code for the app
versionName "1.0" // Version name for the app
}
buildTypes {
release {
minifyEnabled true // Enable code shrinking and obfuscation for release builds
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
flavorDimensions "version" // Product flavor dimension
productFlavors {
free {
dimension "version"
applicationIdSuffix ".free" // Add suffix for free version
}
paid {
dimension "version"
applicationIdSuffix ".paid" // Add suffix for paid version
}
}
}
dependencies {
implementation 'androidx.core:core-ktx:1.6.0' // Core library dependency
implementation 'com.google.android.material:material:1.4.0' // Material design components
implementation 'org.jetbrains.kotlin:kotlin-stdlib-jdk7:1.5.21' // Kotlin standard library
}
Key Concepts of Gradle in Android
- Dependencies Management:
- Gradle simplifies managing third-party libraries and Android SDK dependencies.
- You can include dependencies from various sources, such as Google’s Maven repository, Maven Central, or JCenter.
- Dependencies are declared in the
dependencies
block in the app-levelbuild.gradle
file. Example:
dependencies {
implementation 'com.squareup.retrofit2:retrofit:2.9.0' // Retrofit library for API calls
implementation 'com.google.dagger:dagger:2.38.1' // Dagger library for Dependency Injection
}
- Build Types:
- Gradle supports defining different build types, such as debug and release, with different configurations (e.g., logging or obfuscation).
- In the example above, the release build type enables ProGuard for code minification and obfuscation.
- Product Flavors:
- Gradle allows you to create different versions of your app using product flavors. This is useful when you want to build different variants, such as a free and paid version of your app.
- Each product flavor can have its own configurations, like application ID, version code, or resources.
- Gradle Tasks:
- Gradle builds are composed of tasks, which are unit of work, such as compiling code, testing, and packaging.
- Gradle’s tasks can be run from the command line or executed automatically during the build process.
- Common tasks in Android include
assembleDebug
,assembleRelease
, andbuild
.
- Build Variants:
- Build variants are the combinations of build types and product flavors. For example, with the above configuration, you would have
freeDebug
,freeRelease
,paidDebug
, andpaidRelease
variants. - These variants allow you to customize each version of your app for different configurations, enabling a more flexible development process.
- Build Automation and Continuous Integration:
- Gradle enables automation of the build process. You can configure Gradle to automatically run tests, generate APKs, and deploy to various environments (staging, production) with each commit.
- Continuous Integration (CI) tools like Jenkins, Travis CI, or GitHub Actions use Gradle to automate builds, ensuring the quality and stability of the app.
Gradle Commands for Android
Gradle comes with several useful commands for building and testing Android applications. Below are some common Gradle commands:
- Build the App:
./gradlew build
: Builds the entire project, including all modules../gradlew assembleDebug
: Builds the debug variant of the app../gradlew assembleRelease
: Builds the release variant of the app.
- Clean Project:
./gradlew clean
: Cleans the project by deleting build directories. Useful to fix build issues.
- Run Tests:
./gradlew test
: Runs unit tests defined in your project../gradlew connectedAndroidTest
: Runs the connected Android tests (instrumentation tests).
- Generate APK or AAB:
./gradlew assembleRelease
: Builds the release APK or AAB../gradlew bundleRelease
: Builds an Android App Bundle (AAB) for distribution on the Google Play Store.
- Run the App on an Emulator or Device:
./gradlew installDebug
: Installs the debug version of the APK on a connected device or emulator.
- Sync Gradle:
./gradlew --refresh-dependencies
: Forces Gradle to refresh all dependencies.
Conclusion
Gradle plays a central role in Android app development by automating the build process and managing dependencies, configurations, and resources. It allows developers to create different build variants, manage third-party libraries, and automate testing and deployment. By using Gradle’s build system, Android developers can easily define their build configurations, handle multiple product flavors, and produce optimized APKs and AABs for distribution.
Android Studio integrates seamlessly with Gradle, providing a user-friendly interface for managing builds, dependencies, and configurations. Understanding and mastering Gradle is essential for Android developers who want to build efficient, scalable, and maintainable Android apps.
By leveraging Gradle’s power and flexibility, developers can streamline their development process, enhance collaboration, and ensure the quality and consistency of their Android apps.