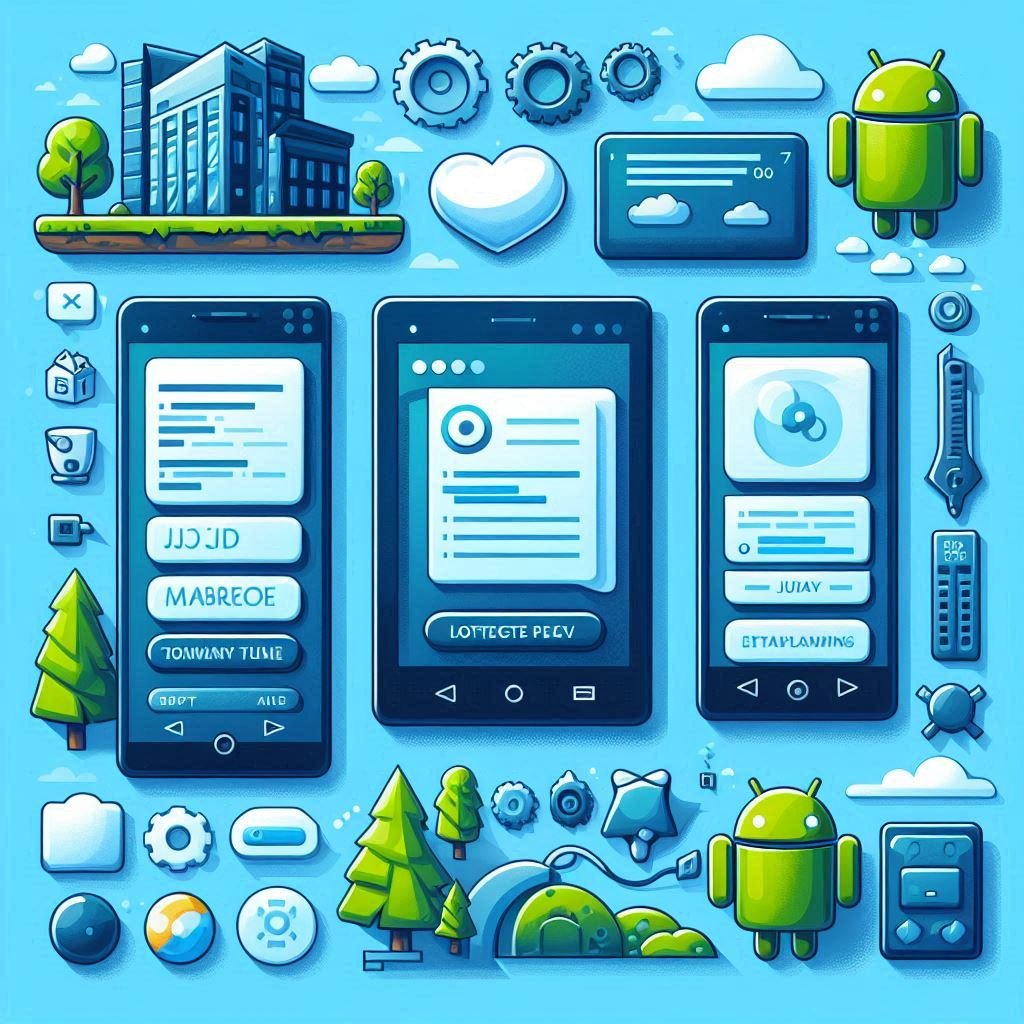
Dialogs in Android App Development Using Java: A Complete Guide
Dialogs are an essential component in Android app development that allows developers to interact with users in a more dynamic and customizable way. Dialogs are typically used to ask users for input, confirm actions, or show informative messages. They are lightweight UI components that appear on top of the current activity to grab the user’s attention.
In Android development with Java, understanding how to work with different types of dialogs, customizing them, and handling user interactions effectively is crucial. This article will explore how to create and manage various types of dialogs in Android apps using Java.
What is a Dialog in Android?
A dialog in Android is a small window that prompts the user to make a decision or enter additional information. Unlike other UI elements such as activities and fragments, dialogs are modal, meaning that they block interaction with the rest of the activity until they are dismissed.
There are several types of dialogs available in Android, including:
- AlertDialog: Used to show simple messages or ask for user confirmation.
- DatePickerDialog: Used to let users select a date.
- TimePickerDialog: Used to let users select a time.
- Custom Dialog: Allows you to create a fully customized dialog with a custom layout and behavior.
Types of Dialogs in Android Using Java
1. AlertDialog
An AlertDialog
is one of the most commonly used dialogs in Android. It can be used to display a simple message or prompt the user to confirm or cancel an action (e.g., “Are you sure you want to delete this item?”).
Example: Basic AlertDialog
import android.content.DialogInterface;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import androidx.appcompat.app.AlertDialog;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button showDialogButton = findViewById(R.id.showDialogButton);
showDialogButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Create and show the alert dialog
AlertDialog.Builder builder = new AlertDialog.Builder(MainActivity.this);
builder.setTitle("Confirm Action")
.setMessage("Are you sure you want to proceed?")
.setCancelable(false) // Prevent dialog from being canceled by tapping outside
.setPositiveButton("Yes", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
// Handle Yes action
}
})
.setNegativeButton("No", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
// Handle No action
}
});
// Create and show the dialog
AlertDialog dialog = builder.create();
dialog.show();
}
});
}
}
- setTitle(): Sets the title of the dialog.
- setMessage(): Sets the message in the dialog.
- setPositiveButton(): Defines an action when the positive button is clicked (e.g., “Yes”).
- setNegativeButton(): Defines an action when the negative button is clicked (e.g., “No”).
This is a basic implementation of an AlertDialog
with “Yes” and “No” options.
2. DatePickerDialog
A DatePickerDialog
allows users to select a date. It is often used when the user needs to pick a date for an event, appointment, or setting a reminder.
Example: DatePickerDialog
import android.app.DatePickerDialog;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
import java.util.Calendar;
public class MainActivity extends AppCompatActivity {
TextView dateText;
Button datePickerButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
dateText = findViewById(R.id.dateText);
datePickerButton = findViewById(R.id.datePickerButton);
datePickerButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Get the current date
final Calendar calendar = Calendar.getInstance();
int year = calendar.get(Calendar.YEAR);
int month = calendar.get(Calendar.MONTH);
int day = calendar.get(Calendar.DAY_OF_MONTH);
// Create a DatePickerDialog
DatePickerDialog datePickerDialog = new DatePickerDialog(MainActivity.this,
(view, selectedYear, selectedMonth, selectedDay) -> {
// Set the selected date to the TextView
dateText.setText(selectedDay + "/" + (selectedMonth + 1) + "/" + selectedYear);
}, year, month, day);
// Show the DatePickerDialog
datePickerDialog.show();
}
});
}
}
- DatePickerDialog: Displays a dialog for selecting a date.
- OnDateSetListener: A callback used to get the selected date when the user confirms.
In this example, the DatePickerDialog
is triggered by a button click, and the selected date is displayed in a TextView
.
3. TimePickerDialog
A TimePickerDialog
is used to let users select a time, which is commonly used for setting alarms, reminders, or event scheduling.
Example: TimePickerDialog
import android.app.TimePickerDialog;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
import java.util.Calendar;
public class MainActivity extends AppCompatActivity {
TextView timeText;
Button timePickerButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
timeText = findViewById(R.id.timeText);
timePickerButton = findViewById(R.id.timePickerButton);
timePickerButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Get the current time
final Calendar calendar = Calendar.getInstance();
int hour = calendar.get(Calendar.HOUR_OF_DAY);
int minute = calendar.get(Calendar.MINUTE);
// Create a TimePickerDialog
TimePickerDialog timePickerDialog = new TimePickerDialog(MainActivity.this,
(view, selectedHour, selectedMinute) -> {
// Set the selected time to the TextView
timeText.setText(selectedHour + ":" + selectedMinute);
}, hour, minute, true);
// Show the TimePickerDialog
timePickerDialog.show();
}
});
}
}
- TimePickerDialog: Displays a dialog for selecting a time.
- OnTimeSetListener: A callback that captures the selected time once the user confirms.
The TimePickerDialog
is used in a similar way to the DatePickerDialog
, with the user selecting a time that is then displayed in a TextView
.
4. Custom Dialog
Sometimes, you might need a more flexible and customized dialog. A custom dialog allows you to use your own layout instead of a predefined one.
Example: Custom Dialog with Custom Layout
import android.app.Dialog;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button showCustomDialogButton = findViewById(R.id.showCustomDialogButton);
showCustomDialogButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Create a custom dialog
Dialog customDialog = new Dialog(MainActivity.this);
customDialog.setContentView(R.layout.custom_dialog_layout);
// Initialize custom dialog views
EditText userInput = customDialog.findViewById(R.id.userInput);
Button submitButton = customDialog.findViewById(R.id.submitButton);
// Handle the submit button click
submitButton.setOnClickListener(v1 -> {
String inputText = userInput.getText().toString();
// Do something with the input
customDialog.dismiss();
});
// Show the custom dialog
customDialog.show();
}
});
}
}
In this example, a custom layout (custom_dialog_layout.xml
) is used for the dialog. This layout can contain any views you wish, such as EditText
, TextView
, Buttons
, etc.
- Dialog.setContentView(): Sets the layout resource for the custom dialog.
- You can define interactions with views inside the custom dialog, like submitting user input.
Conclusion
Dialogs are a versatile and essential part of Android app development, providing a way for users to interact with your app in a simple yet effective manner. Whether you are using a basic AlertDialog
, a DatePickerDialog
, or building a fully custom dialog, Android provides multiple options to handle user input and display messages.
By using dialogs in Android, you can improve the user experience by providing feedback, confirming actions, or collecting input in an intuitive way. Understanding how to create and customize dialogs using Java will help you implement interactive and responsive features
in your Android applications.