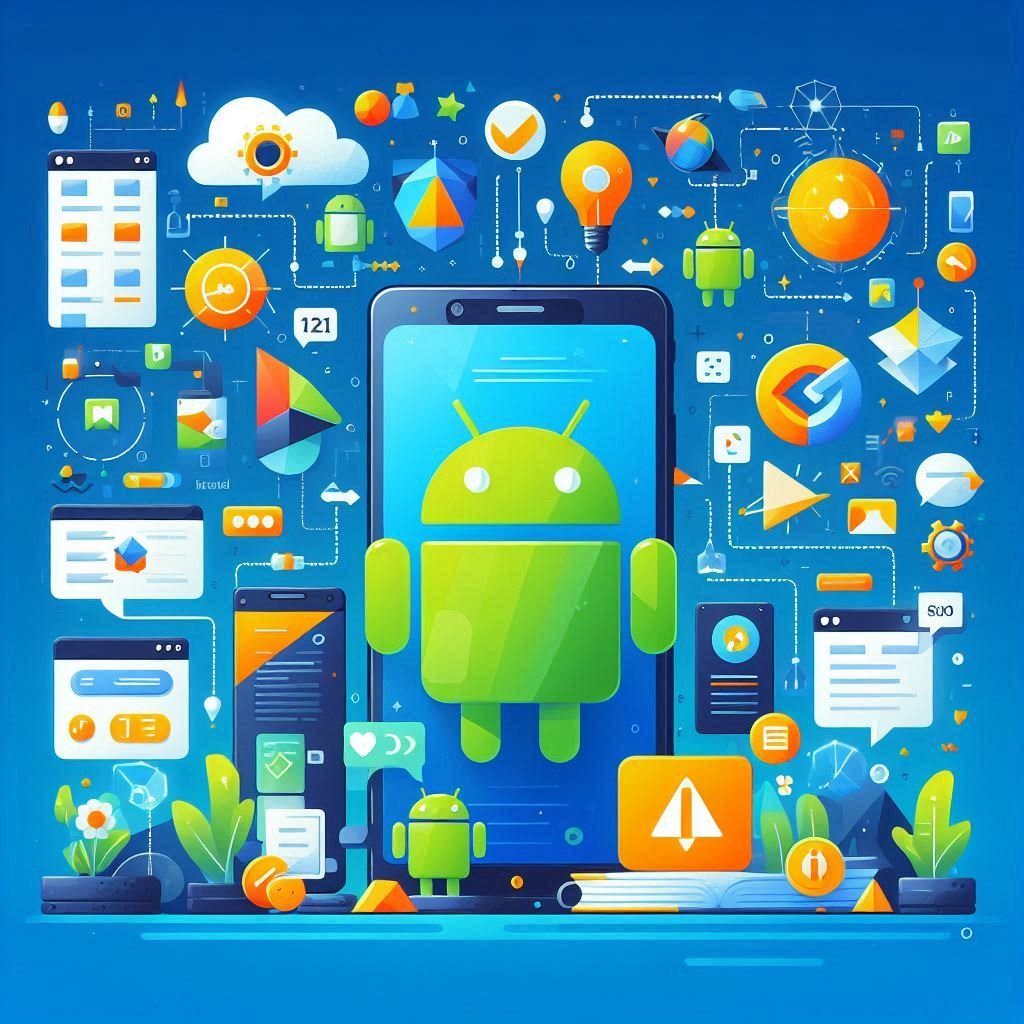
How to Connect Firebase to Your Android App in Java
Firebase is a powerful platform developed by Google for mobile and web app development, offering a variety of tools and services that simplify backend tasks. In Android app development, Firebase helps with functions like user authentication, real-time databases, cloud storage, and more. In this article, we will guide you through the steps of connecting Firebase to your Android app using Java.
Step 1: Set Up Firebase in Your Android Project
Before you can use Firebase in your Android app, you need to set up a Firebase project and configure your app to use Firebase services.
1.1. Create a Firebase Project
To get started, you need to create a Firebase project:
- Go to the Firebase Console: Visit Firebase Console.
- Click on “Add Project”: You will be prompted to create a new project. Follow the steps to name your project and choose whether to enable Google Analytics (this is optional).
- Complete the Setup: Once the project is created, Firebase will automatically redirect you to the project dashboard.
1.2. Register Your Android App with Firebase
Next, you need to link your Android app with your Firebase project.
- Go to the Firebase Console and select your newly created Firebase project.
- Click on the Android Icon to add an Android app to your Firebase project.
- Enter Your Android Package Name: This is the unique identifier for your app, which can be found in your
AndroidManifest.xml
file orbuild.gradle
file underapplicationId
. - Download the
google-services.json
file: This file contains essential configuration details for Firebase. Place this file in your Android project’sapp/
folder (inside thesrc/
directory).
1.3. Add the Firebase SDK to Your Project
Now you need to include Firebase SDK dependencies in your project.
- Modify Project-level
build.gradle
:
Open the project-levelbuild.gradle
file, and add the following classpath in thedependencies
section:
buildscript {
repositories {
google() // Google's Maven repository
mavenCentral()
}
dependencies {
classpath 'com.google.gms:google-services:4.3.15' // Check for the latest version
}
}
- Modify App-level
build.gradle
:
Open the app-levelbuild.gradle
file and apply the Google services plugin at the bottom:
apply plugin: 'com.google.gms.google-services'
Also, include Firebase dependencies for the services you plan to use. For example, for Firebase Authentication and Firebase Realtime Database, add:
dependencies {
implementation 'com.google.firebase:firebase-auth:21.0.8' // Firebase Authentication
implementation 'com.google.firebase:firebase-database:20.0.5' // Firebase Realtime Database
}
- Sync Gradle:
Click Sync Now to sync your project with the Firebase dependencies.
Step 2: Initialize Firebase in Your Android App
Firebase is initialized automatically when the app starts, but you can explicitly initialize it in your app’s MainActivity.java
(or any other activity where you want to use Firebase services).
In your MainActivity.java
:
import android.os.Bundle;
import androidx.appcompat.app.AppCompatActivity;
import com.google.firebase.database.FirebaseDatabase;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Firebase is automatically initialized when the app starts
FirebaseDatabase database = FirebaseDatabase.getInstance();
// Now, you can start using Firebase services
}
}
At this point, Firebase is initialized and ready to use within your app.
Step 3: Using Firebase Services in Your Android App
Now that Firebase is connected, you can start using Firebase features such as Authentication, Realtime Database, and Cloud Storage. Let’s go over two of the most common services:
3.1. Firebase Authentication
Firebase Authentication helps manage user sign-ups and sign-ins securely. Below is an example of implementing Email/Password authentication.
- Enable Authentication in Firebase Console:
- In the Firebase Console, go to the Authentication section.
- Under the Sign-in method tab, enable the Email/Password sign-in provider.
- Implementing Email/Password Authentication in Java:
import android.os.Bundle;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
import com.google.firebase.auth.FirebaseAuth;
public class LoginActivity extends AppCompatActivity {
private EditText emailEditText, passwordEditText;
private Button loginButton;
private FirebaseAuth mAuth;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
emailEditText = findViewById(R.id.emailEditText);
passwordEditText = findViewById(R.id.passwordEditText);
loginButton = findViewById(R.id.loginButton);
mAuth = FirebaseAuth.getInstance();
loginButton.setOnClickListener(v -> {
String email = emailEditText.getText().toString();
String password = passwordEditText.getText().toString();
if (email.isEmpty() || password.isEmpty()) {
Toast.makeText(this, "Email and password required", Toast.LENGTH_SHORT).show();
return;
}
// Sign in using Firebase Authentication
mAuth.signInWithEmailAndPassword(email, password)
.addOnCompleteListener(this, task -> {
if (task.isSuccessful()) {
// Successfully signed in
Toast.makeText(LoginActivity.this, "Login successful!", Toast.LENGTH_SHORT).show();
} else {
// Failed to sign in
Toast.makeText(LoginActivity.this, "Authentication failed.", Toast.LENGTH_SHORT).show();
}
});
});
}
}
In this example, the user is authenticated with their email and password. If successful, the app navigates to a new screen; otherwise, an error message is displayed.
3.2. Firebase Realtime Database
Firebase Realtime Database allows you to store and sync data in real-time across clients. Here’s how you can use it to store and retrieve data.
- Enable Firebase Realtime Database:
- In the Firebase Console, go to Database.
- Choose Realtime Database and click Create Database.
- Set up the database rules (for testing, you can set read/write permissions to
true
).
- Writing Data to Firebase Realtime Database:
import android.os.Bundle;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
import com.google.firebase.database.DatabaseReference;
import com.google.firebase.database.FirebaseDatabase;
public class MainActivity extends AppCompatActivity {
private EditText nameEditText;
private Button saveButton;
private FirebaseDatabase database;
private DatabaseReference databaseReference;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
nameEditText = findViewById(R.id.nameEditText);
saveButton = findViewById(R.id.saveButton);
database = FirebaseDatabase.getInstance();
databaseReference = database.getReference("users");
saveButton.setOnClickListener(v -> {
String name = nameEditText.getText().toString();
if (name.isEmpty()) {
Toast.makeText(MainActivity.this, "Please enter a name", Toast.LENGTH_SHORT).show();
return;
}
// Create a unique user ID
String userId = databaseReference.push().getKey();
User user = new User(userId, name);
// Write the user data to the Realtime Database
databaseReference.child(userId).setValue(user)
.addOnCompleteListener(task -> {
if (task.isSuccessful()) {
Toast.makeText(MainActivity.this, "Data saved successfully", Toast.LENGTH_SHORT).show();
} else {
Toast.makeText(MainActivity.this, "Failed to save data", Toast.LENGTH_SHORT).show();
}
});
});
}
}
In this example, user data is stored in Firebase’s Realtime Database under the users
node. The data can be retrieved and displayed on other devices connected to the app in real-time.
Step 4: Test Your Firebase Integration
- Test Authentication:
- Test if users can successfully sign up and sign in using Firebase Authentication.
- Use the Firebase Console to monitor users and verify that authentication works.
- Test Realtime Database:
- Check the Firebase Console to see if data is correctly being saved to the Realtime Database.
- Test reading and writing data in real-time by running your app on different devices.
Conclusion
Connecting Firebase to your Android app in Java is a straightforward process that involves setting up Firebase, adding necessary dependencies, and implementing Firebase features like Authentication and Realtime Database. With Firebase, you gain access to a variety of backend services that make it easier to build robust, scalable apps.
By following the steps in this guide, you can easily integrate Firebase into your Android projects and start using its powerful features to enhance your app’s functionality.