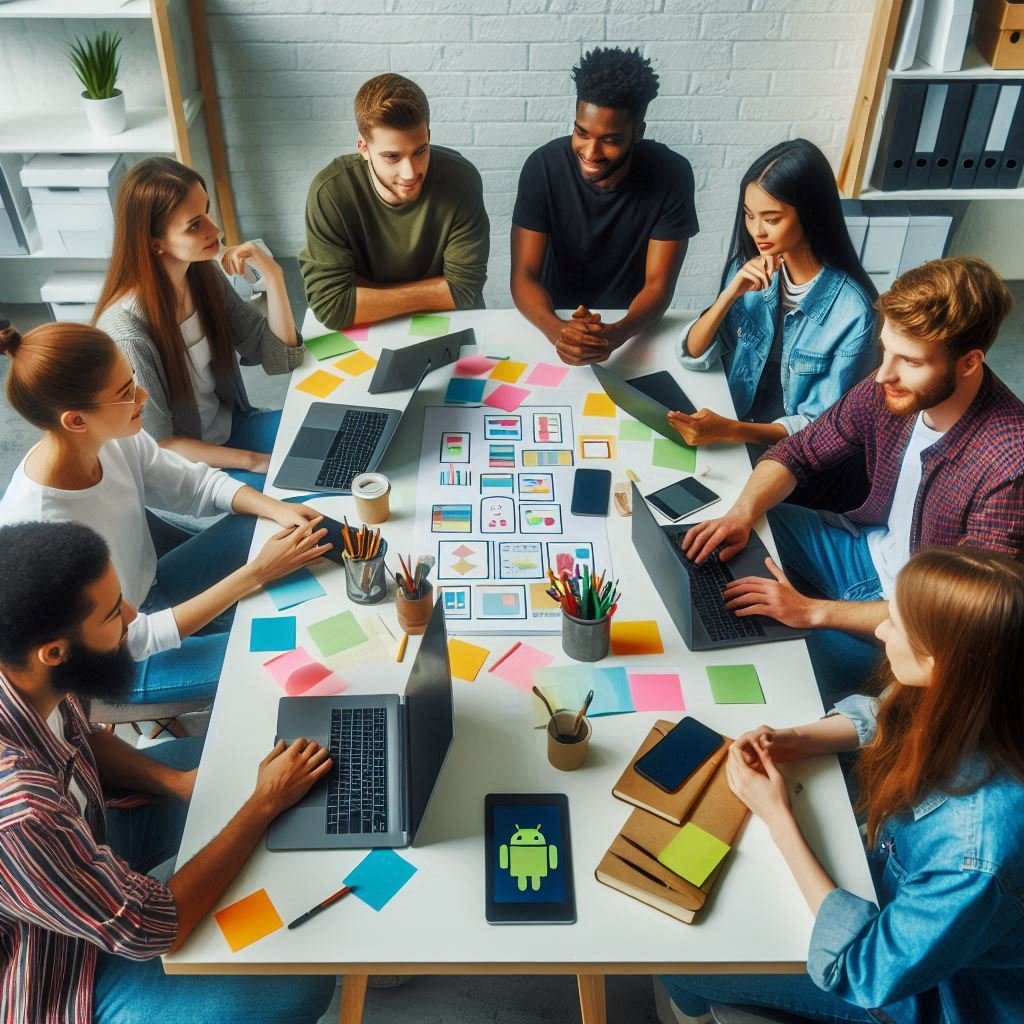
Manifest in Android App Development: A Complete Guide
The AndroidManifest.xml file is one of the most important components in Android app development. It provides essential information about the app to the Android operating system and defines how the app should behave. Without this file, an Android app cannot function because the system needs the manifest to know about the app’s components, permissions, and other configurations.
In this article, we’ll explore what the Manifest is, why it’s important, and how to properly configure it in your Android app development.
What is the AndroidManifest.xml File?
The AndroidManifest.xml file is an XML file located in the root directory of your Android project. It provides essential information about the application, such as:
- Declaring the components of the app (activities, services, broadcast receivers, and content providers).
- Specifying permissions required by the app.
- Defining hardware and software features the app requires.
- Setting the app’s launch behavior, theme, and other configurations.
The manifest file is processed by the Android build system to produce an APK (Android Package) that the Android operating system can install and run.
Structure of the AndroidManifest.xml File
Here’s the basic structure of the AndroidManifest.xml file:
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.myapp">
<application
android:label="My App"
android:icon="@mipmap/ic_launcher">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Key Elements of the Manifest
Here’s a breakdown of the primary elements that you’ll find in the AndroidManifest.xml file:
<manifest>
: This is the root element of the manifest file. It includes thepackage
attribute, which defines the unique identifier for the app (i.e., the package name).<application>
: The<application>
element defines the app’s settings, including the app’s name, icon, theme, and components. Inside this tag, you’ll define the different components of your app, such as activities, services, broadcast receivers, and content providers.<activity>
: An activity represents a single screen with a user interface. The<activity>
element defines an activity in your app and includes various attributes likeandroid:name
(the class name of the activity) and<intent-filter>
(to define which intents the activity can respond to). The example above shows how an activity is declared, and theMAIN
action andLAUNCHER
category are specified to set this activity as the entry point (the main activity) of the app.<intent-filter>
: The<intent-filter>
is used to specify what types of intents an activity can handle. For example, in the above code, it specifies that theMainActivity
should be launched when the user starts the app.<service>
: A service runs in the background and performs long-running operations. You define a service in the manifest with the<service>
tag. For example, if your app has a background service that downloads files, it would be declared in the manifest file.<receiver>
: This element is used to declare broadcast receivers. A broadcast receiver is a component that listens for specific system-wide broadcasts, such as network changes, battery status, or custom messages sent by the app itself.<provider>
: Content providers manage shared data and provide access to it. The<provider>
element allows your app to share its data with other apps or access data from other apps.- Permissions: Permissions in the manifest are essential to declare what the app can or cannot do, like accessing the internet, reading contacts, or using the camera. You declare permissions using the
<uses-permission>
tag. For example:
<uses-permission android:name="android.permission.INTERNET" />
This grants the app access to the internet.
Key Features Defined in the AndroidManifest.xml File
- Application Components:
The manifest file declares all app components, which are essential for the app to function. These include:
- Activities: Represent the UI components or screens.
- Services: Handle background tasks and operations.
- Broadcast Receivers: Listen to and respond to system-wide events.
- Content Providers: Share and manage data with other applications.
- Permissions:
Permissions define what parts of the Android system or other apps your app can access. Permissions are declared in the manifest file to request access to sensitive data or features, like the camera, GPS, or internet access. Some common permissions include:
INTERNET
ACCESS_FINE_LOCATION
READ_EXTERNAL_STORAGE
For example, if your app needs to access the device’s camera, you would add:
<uses-permission android:name="android.permission.CAMERA" />
- App Configuration:
- App Theme: You can set the default theme for your app using the
android:theme
attribute in the<application>
tag. - App Icon: You can specify the app’s icon using
android:icon
. - App Label: This attribute sets the app’s name, which appears on the home screen or app launcher.
- Handling Intent Filters:
- You can define which intent your app’s components can respond to. For example, if an activity is meant to handle a particular action or URL, the corresponding
<intent-filter>
is used to declare the supported actions and categories. For example:
<activity android:name=".BrowserActivity">
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<data android:scheme="http" android:host="www.example.com" />
</intent-filter>
</activity>
- Declaring App Features:
- You can specify which features your app requires by using the
<uses-feature>
tag. This is helpful for devices that don’t support certain features. For example, if your app requires a camera, you can add:
<uses-feature android:name="android.hardware.camera" android:required="true" />
Essential Uses of the AndroidManifest.xml File
- Setting the Main Activity:
The main activity of your app is defined in the manifest, and it’s where the app will begin executing when it is launched. This is the activity that the system should use to start the app. Example:
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
- Requesting Permissions:
If your app requires access to system resources or sensitive data, you must declare the necessary permissions in the manifest. For example:
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
- Declaring Services and Broadcast Receivers:
If your app needs to run background services or handle broadcasts (system-wide messages), these components need to be declared in the manifest.
Example of declaring a service:
<service android:name=".MyService" />
- Defining App Themes:
You can specify the default theme for your app, which will define the look and feel of the app’s UI.
Example:
<application
android:theme="@style/AppTheme">
</application>
- Handling Different Android Versions:
The manifest helps ensure that your app behaves correctly across different Android versions. You can specify features or configurations required for different versions of Android using theminSdkVersion
,targetSdkVersion
, anduses-sdk
elements.
Best Practices for AndroidManifest.xml
- Keep Permissions Minimal: Only request permissions that are absolutely necessary for your app’s functionality. Asking for too many permissions can deter users from installing your app.
- Use Proguard to Minimize Code Exposure: If you’re using Proguard (for code minification), make sure your
AndroidManifest.xml
includes the necessary entries to prevent critical code and components from being stripped during the build process. - Declare Intents Clearly: Make sure that your app’s intents are well-defined and correctly specified so that the right components respond to the right events.
- Versioning and Compatibility: Always specify your app’s
minSdkVersion
andtargetSdkVersion
correctly to ensure compatibility with the right Android versions. This is especially important as Android versions evolve and deprecate old APIs.
Conclusion
The AndroidManifest.xml file is crucial in Android app development, as it provides the system with all necessary information about the app’s components, permissions, configurations, and app behavior. It acts as the heart of the app, where key attributes such as activities, services, permissions, and more are declared. Understanding how to configure and use the AndroidManifest.xml
file properly is essential for building well-functioning and secure Android applications.