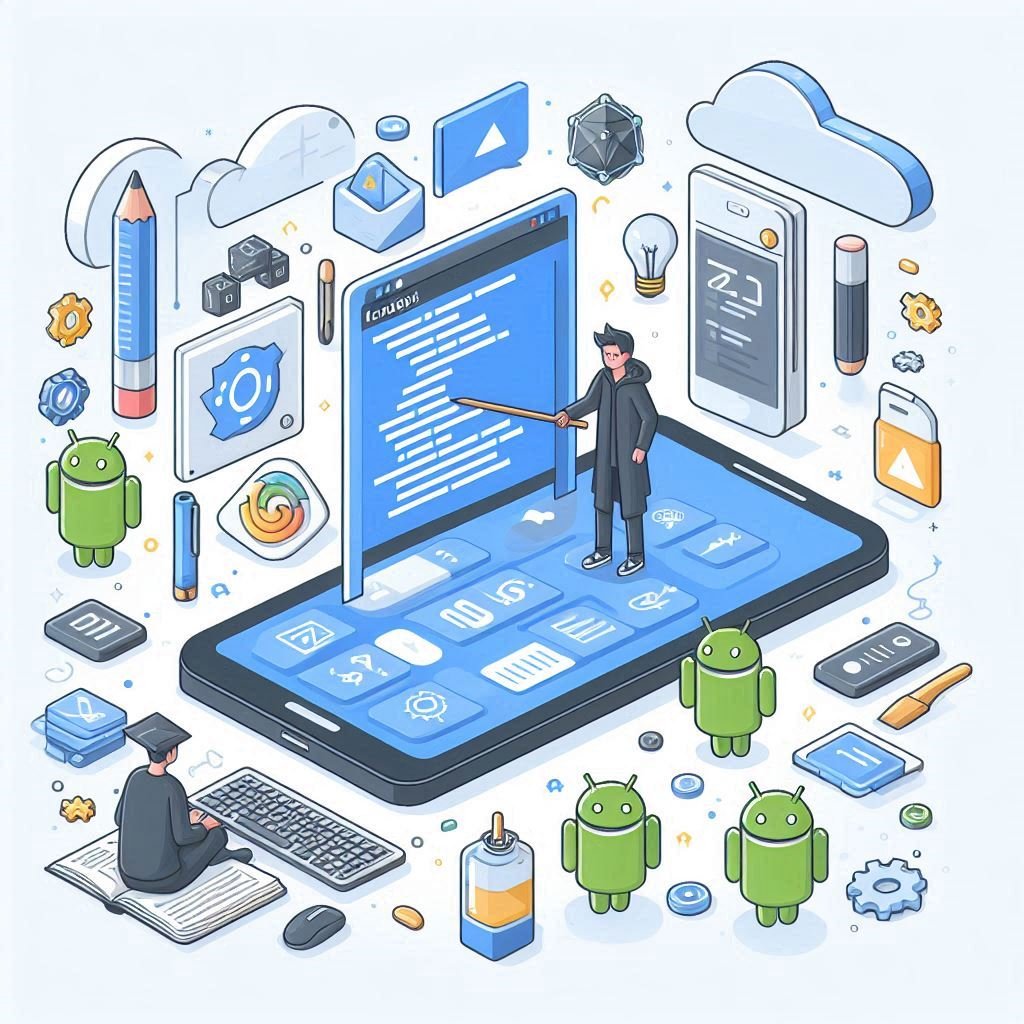
Intent in Android App Development Using Java: A Complete Guide
In Android app development, Intents play a crucial role as they enable communication between various components of an app, such as activities, services, and broadcast receivers. An Intent can be thought of as a message that informs the Android system about an action to perform, such as opening a new activity or sharing data with another app. This guide will cover everything you need to know about Intents in Android development using Java.
What is an Intent?
In Android, an Intent is a messaging object that facilitates communication between components within an app or even across apps. Intents allow you to:
- Start a new activity.
- Start a service.
- Broadcast a message to other components.
- Transfer data between activities.
Intents can be categorized into two main types:
- Explicit Intent: Used to launch a specific activity or service within the same app.
- Implicit Intent: Used to request an action from other apps or components capable of handling the intent.
Types of Intents
1. Explicit Intent
An Explicit Intent specifies the exact component (activity, service) that should handle the intent. This is useful for internal app navigation, such as moving from one activity to another.
Example:
Intent intent = new Intent(CurrentActivity.this, TargetActivity.class);
startActivity(intent);
In this example:
CurrentActivity.this
is the context of the current activity.TargetActivity.class
specifies the activity to open.
2. Implicit Intent
An Implicit Intent does not specify a particular component but declares a general action to be performed, allowing other apps or components to handle it.
Example:
Intent intent = new Intent(Intent.ACTION_VIEW);
intent.setData(Uri.parse("https://www.google.com"));
startActivity(intent);
Here:
Intent.ACTION_VIEW
indicates a general action to view content.setData(Uri.parse("https://www.google.com"))
provides the data (a URL) to be viewed.
This example would open a web browser to view the specified URL, as multiple apps can handle the action of viewing a webpage.
Using Intent to Start Activities
To navigate between activities, you use Intents in the following way:
- Create an Intent object, specifying the current activity and the target activity.
- Start the activity with the
startActivity()
method.
Example:
Intent intent = new Intent(MainActivity.this, SecondActivity.class);
startActivity(intent);
In this example:
MainActivity.this
refers to the context of the current activity.SecondActivity.class
is the activity you want to start.
Passing Data Between Activities Using Intents
One of the most common uses of Intents is passing data between activities. You can use putExtra()
to add data to the intent and getStringExtra()
, getIntExtra()
, etc., to retrieve the data in the target activity.
Passing Data Example:
Intent intent = new Intent(MainActivity.this, SecondActivity.class);
intent.putExtra("MESSAGE", "Hello from MainActivity!");
startActivity(intent);
Retrieving Data in Target Activity:
// In SecondActivity
String message = getIntent().getStringExtra("MESSAGE");
TextView textView = findViewById(R.id.textView);
textView.setText(message);
Here:
putExtra("MESSAGE", "Hello from MainActivity!")
adds a string data with the key “MESSAGE” to the intent.getStringExtra("MESSAGE")
retrieves the data inSecondActivity
.
Starting an Activity for a Result
Sometimes, you may want to receive a result from an activity you start. For example, you might open a contact picker and get the selected contact information back. To achieve this, use startActivityForResult()
in the initiating activity and handle the result in onActivityResult()
.
Starting Activity with Result:
Intent intent = new Intent(MainActivity.this, SecondActivity.class);
startActivityForResult(intent, REQUEST_CODE);
Handling the Result:
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == REQUEST_CODE && resultCode == RESULT_OK) {
String result = data.getStringExtra("result");
TextView textView = findViewById(R.id.textView);
textView.setText(result);
}
}
Setting Result in Target Activity:
Intent resultIntent = new Intent();
resultIntent.putExtra("result", "Result from SecondActivity");
setResult(RESULT_OK, resultIntent);
finish();
In this example:
startActivityForResult()
startsSecondActivity
and expects a result back.setResult()
inSecondActivity
sets the result before finishing the activity.onActivityResult()
inMainActivity
receives and handles the result.
Common Intent Actions
Android provides many built-in actions for Implicit Intents to facilitate common tasks:
- Open a Web Page:
Intent intent = new Intent(Intent.ACTION_VIEW); intent.setData(Uri.parse("https://www.example.com")); startActivity(intent);
- Dial a Number:
Intent intent = new Intent(Intent.ACTION_DIAL); intent.setData(Uri.parse("tel:123456789")); startActivity(intent);
- Send an Email:
Intent intent = new Intent(Intent.ACTION_SENDTO); intent.setData(Uri.parse("mailto:example@example.com")); intent.putExtra(Intent.EXTRA_SUBJECT, "Subject"); intent.putExtra(Intent.EXTRA_TEXT, "Email message text"); startActivity(intent);
- Capture a Photo:
Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE); startActivityForResult(intent, REQUEST_IMAGE_CAPTURE);
- Select a Contact:
java Intent intent = new Intent(Intent.ACTION_PICK, ContactsContract.Contacts.CONTENT_URI); startActivityForResult(intent, REQUEST_SELECT_CONTACT);
Broadcast Intents
Broadcast Intents are another powerful feature in Android that allow components to send messages to other parts of the system or other apps. Broadcasts can be system-wide or limited to your app.
- Sending a Broadcast:
Intent intent = new Intent("com.example.CUSTOM_ACTION"); sendBroadcast(intent);
- Receiving a Broadcast:
Create aBroadcastReceiver
to handle incoming broadcasts.java public class MyBroadcastReceiver extends BroadcastReceiver { @Override public void onReceive(Context context, Intent intent) { // Handle the broadcast } }
Register the receiver in AndroidManifest.xml
or dynamically in code.
Intent Filters
Intent Filters allow an activity, service, or broadcast receiver to respond to implicit intents. By specifying filters in AndroidManifest.xml
, you can declare which actions, categories, or data types your component can handle.
Example:
<activity android:name=".SecondActivity">
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<data android:mimeType="text/plain" />
</intent-filter>
</activity>
In this example, SecondActivity
can handle intents with the action VIEW
and data type text/plain
.
Conclusion
Intents are essential for app navigation, data sharing, and communication between components. They enable a flexible, powerful system that allows users to experience a seamless flow across an app and even interact with other apps. Whether you’re using explicit intents for internal navigation or implicit intents to leverage other apps’ features, understanding and implementing intents will significantly enhance your app’s functionality.